Exploring Custom Cryptography Techniques
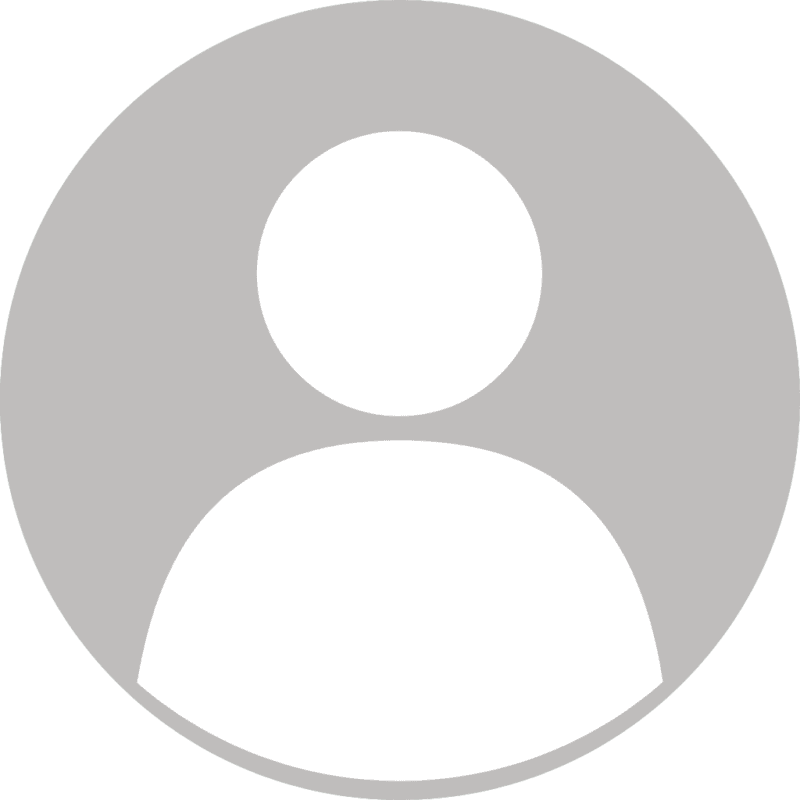
Custom cryptography surround a range of techniques for securing data beyond established cryptographic standards. While often viewed with caution due to potential security risks, custom cryptography can offer unique advantages in specific scenarios. This article explores various custom cryptography approaches, with a focus on "in-house cryptography" and its real-world applications.
Types of Custom Cryptography
- Modified Standard Algorithms: This involves adapting existing algorithms like AES or RSA by changing parameters or adding functionalities. While seemingly straightforward, even minor modifications can introduce vulnerabilities if not carefully designed and analyzed.
- Proprietary Algorithms: Companies may develop entirely new encryption algorithms from scratch. This approach demands exceptional cryptographic expertise, as even slight errors can compromise security. Careful testing and peer review are crucial for such algorithms.
- Customized Cryptographic Systems: Standard algorithms can be combined to create custom cryptographic systems for specific use cases. This approach influences the strengths of established algorithms while addressing unique application requirements.
In-House Cryptography
In-house cryptography refers to the development of cryptographic algorithms within an organization for its exclusive use. While it offers potential benefits like:
- Performance Optimization: Custom algorithms can be customized for specific hardware or performance needs.
- Integration with Existing Systems: Seamless integration with existing proprietary systems might be achieved.
However, significant challenges exist:
- Security Risks: In-house algorithms often lack careful testing and public scrutiny that established standards undergo. This can lead to vulnerabilities.
- Maintenance Burden: Custom algorithms require ongoing maintenance and security updates, which can be a significant resource drain.
- Transparency Concerns: Security through obscurity (relying on secrecy of the algorithm) is generally discouraged. Trust is often better established through open, well-reviewed standards.[Wikipedia]
Example: Homophonic Substitution with Symbol Rotation (LUA)
Here's a basic example in LUA demonstrating a custom approach combining homophonic substitution (replacing characters with multiple alternatives) and symbol rotation (shifting symbols by a specific value or randomly):
function encrypt(text, key)
local alphabet = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ !1234567890"
local shifted_alphabet = string.sub(alphabet, key + 1, string.len(alphabet)) .. string.sub(alphabet, 1, key)
local mapping = {}
for i = 1, string.len(alphabet) do
mapping[string.sub(alphabet, i, i)] = string.sub(shifted_alphabet, i, i)
end
local ciphertext = ""
for i = 1, string.len(text) do
local char = string.sub(text, i, i)
print(mapping[char])
if ciphertext~= nil then
ciphertext = ciphertext .. mapping[char] or char
else
ciphertext = mapping[char] or char
end
end
return ciphertext
end
local message = "This is a secret message! 123"
local key = 3
local encrypted_text = encrypt(message, key)
print("Original Message:", message)
print("Encrypted Text:", encrypted_text)
The if-else statement is needed because empty strings are considered nil in Lua, and nil values won't be concatenated with other strings.
if ciphertext~= nil then
ciphertext = ciphertext .. mapping[char] or char
else
ciphertext = mapping[char] or char
end
Also, note that the 'alphabet' variable inside the function defines the supported characters. If our message includes a character like '#', which isn't in the 'alphabet', an error will occur when the function tries to find it in the mapping.
Now, if we run the code, the following output will be printed:
Encrypted Text: Wklv2lv2d2vhfuhw2phvvdjh32456
The plenty of '2's in the encrypted text suggests they represent spaces (Spaces are dealt with as any other character). We can reduce this vulnerability by using Symbol Rotation, which would make the encrypted text appear more random & harder to decrypt it would be just like below:
Encrypted Text: vhsW6uhJiv6w7lfv3hsdj1p6v8h62
One way to implement symbol rotation is to assign 5 unique characters to represent each letter in your supported character set. To use a wider range of symbols, you could use UTF-8 characters. Store these characters in a table, and determine the representation based on their position. For example, myTable[5] to myTable[10] could all represent the letter 'a'.
Why It Could Be Hard to Crack
This example combines two custom techniques, making it more complex to crack compared to a simple substitution cipher. However, it's important to note that this is a basic illustration, and a determined attacker with advanced cryptanalysis techniques could potentially break it.
Use Cases for Custom Cryptography
Custom cryptography might be considered for:
- Internal communication within a highly secure organization with significant cryptographic expertise on staff.
- Proprietary data formats where tight integration with existing systems is crucial.
However, for most data security needs, established cryptographic standards offer a more reliable and well-vetted approach.
Conclusion
Custom cryptography can open up new possibilities for innovation, but it must be done with caution and an in-depth understanding of the risks of security involved. Established cryptographic standards are frequently a more secure and possible answer for most data protection requirements.
Comments